LIMITED TIME OFFER
Replace all of these

with a single tool for just $49 per month for your entire team
UNLIMITED USERS
UNLIMITED PROJECTS
UNLIMITED CHATS
UNLIMITED DOCS
UNLIMITED STORAGE
AND MORE..
A Comprehensive Guide to Code Refactoring in Software Development
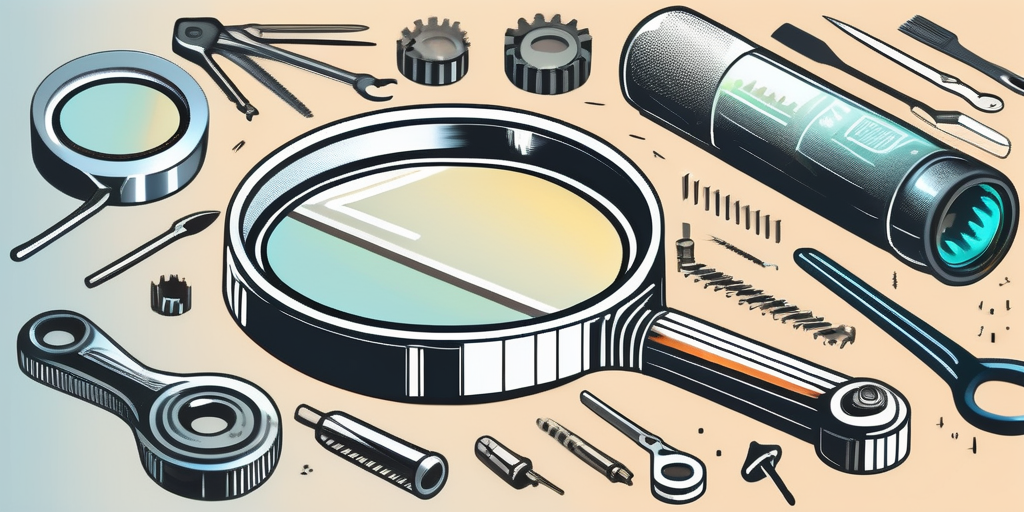
Code refactoring is an essential aspect of software development that allows developers to improve the quality and maintainability of their code. By restructuring and optimizing existing code without changing its external behavior, refactoring enables teams to enhance software performance, reduce complexity, and boost productivity. In this comprehensive guide, we will explore the definition, principles, steps, techniques, tools, challenges, and benefits of code refactoring. So let’s dive into the world of code refactoring and uncover its secrets to empower you as a software developer.
Understanding Code Refactoring
Definition and Importance of Code Refactoring
Code refactoring refers to the process of modifying existing code to improve its internal structure, design, or performance without altering its external functionality. It involves making changes that enhance code readability, reduce complexity, and eliminate technical debt. While code refactoring may seem like an additional effort, it plays a crucial role in software development projects.
Importantly, code refactoring allows developers to keep their codebase clean, maintainable, and scalable. It improves the overall quality of the software, making it easier to add new features and fix bugs. By refactoring regularly, development teams also ensure that their codebase remains adaptable to changing requirements and emerging technologies.
The Role of Code Refactoring in Software Development
Code refactoring is not limited to optimizing code quality. It also enables developers to align their code with best practices and industry standards. Additionally, refactoring plays a vital role in the long-term success of software projects. It helps prevent technical debt from accumulating and avoids the infamous “Big Ball of Mud” phenomenon.
Moreover, refactoring allows developers to address performance bottlenecks, enhance software maintainability, and improve team collaboration. By prioritizing code refactoring, organizations foster a culture of continuous improvement and ensure the sustainability of their software products.
Furthermore, code refactoring can lead to improved code reusability. When developers refactor their code, they often identify opportunities to extract reusable components or modules. These reusable components can then be used in other parts of the software or even in future projects. This not only saves development time but also promotes consistency across different codebases.
In addition, code refactoring can have a positive impact on the overall development process. By regularly refactoring their code, developers become more familiar with the codebase and gain a deeper understanding of its intricacies. This familiarity allows them to identify potential issues or areas for improvement more quickly, leading to more efficient development cycles.
Furthermore, code refactoring can contribute to improved code maintainability. As software projects evolve and grow, maintaining the codebase becomes increasingly challenging. Refactoring helps to keep the codebase organized and manageable, making it easier for developers to navigate and make changes. This, in turn, reduces the risk of introducing bugs or unintended side effects when modifying the code.
Lastly, code refactoring can have a positive impact on team collaboration. When developers regularly refactor their code, they create a shared understanding of the codebase among team members. This shared understanding facilitates effective communication, knowledge sharing, and collaboration. It also reduces the dependency on individual developers, making it easier for team members to work together on different parts of the codebase.
The Principles of Code Refactoring
The Rule of Three in Refactoring
The “Rule of Three” is a fundamental principle in code refactoring. It states that if you find yourself duplicating a piece of code in three or more places, it’s time to refactor it into a reusable component or method. By eliminating duplication, developers improve code maintainability and reduce the risk of introducing errors when changes are required.
Applying the Rule of Three allows developers to create modular, reusable, and maintainable code, leading to increased productivity and codebase stability.
Let’s take a closer look at why the Rule of Three is such an important concept in code refactoring. Imagine you’re working on a large project with multiple developers. As the project grows, so does the complexity of the codebase. Without proper refactoring, you may start to notice duplicated code scattered throughout the project. This duplication not only makes the code harder to maintain but also increases the chances of introducing bugs.
By following the Rule of Three, you can proactively address code duplication before it becomes a significant issue. When you identify a piece of code that appears in three or more places, you can refactor it into a reusable component or method. This not only eliminates the duplication but also promotes code reusability and modularity.
The Don’t Repeat Yourself (DRY) Principle
The Don’t Repeat Yourself (DRY) principle is closely related to the Rule of Three. It emphasizes the importance of avoiding code duplication by abstracting common functionality into reusable components. The DRY principle promotes code simplicity, clarity, and maintainability.
By adhering to DRY, developers can minimize the chances of introducing bugs, reduce overall codebase complexity, and make future modifications and enhancements more efficient.
Let’s delve deeper into the benefits of following the DRY principle. When you encounter duplicated code, it’s not just a matter of aesthetics or code cleanliness. Duplicated code can lead to a maintenance nightmare. Imagine having to make a change to a piece of code that appears in multiple places. Without adhering to the DRY principle, you would need to update each occurrence individually, increasing the chances of missing a spot or introducing inconsistencies.
By abstracting common functionality into reusable components, you create a single source of truth. Any changes or updates made to the reusable component will automatically reflect in all the places where it is used. This not only saves time and effort but also ensures consistency throughout the codebase.
Steps in the Code Refactoring Process
Identifying Areas for Refactoring
The first step in the code refactoring process is identifying areas of the codebase that could benefit from refactoring. This can be done through code reviews, analysis of performance bottlenecks, or by addressing specific pain points raised by the development team.
By conducting a thorough assessment, developers can prioritize refactoring tasks and create a roadmap for improving the codebase.
During the assessment, it is essential to consider various factors that may indicate the need for refactoring. These factors include code complexity, duplication, poor naming conventions, and lack of modularity. By carefully analyzing these aspects, developers can pinpoint specific areas that require attention.
For example, if a code review reveals a section of code that is difficult to understand and maintain due to its complexity, it may be a prime candidate for refactoring. Similarly, if multiple modules in the codebase share similar functionality, refactoring can help consolidate and streamline the code, improving its overall quality.
Prioritizing Refactoring Tasks
Once areas for refactoring have been identified, it’s important to prioritize the tasks based on their impact on software quality and the level of effort required. Prioritizing refactoring tasks ensures that the most critical code sections are improved first, leading to immediate benefits.
Furthermore, establishing clear priorities helps allocate development resources effectively and ensures a structured and systematic approach to code refactoring.
When prioritizing refactoring tasks, developers should consider the potential impact on the software’s performance, maintainability, and scalability. For example, if a particular code section is causing significant performance bottlenecks, it should be given higher priority to optimize the overall system efficiency.
Additionally, the level of effort required for each refactoring task should be taken into account. Some tasks may involve minor code adjustments, while others may require significant architectural changes. By considering the effort required, developers can plan and allocate resources accordingly, ensuring a smooth and efficient refactoring process.
Common Code Refactoring Techniques
Reducing Code Complexity
Code complexity is one of the biggest barriers to code understandability and maintainability. Refactoring techniques such as extracting methods, eliminating nested conditionals, and simplifying complex expressions can significantly reduce code complexity.
By breaking down complex logic into smaller, well-defined functions, developers make code easier to read and understand, leading to improved code quality and enhanced maintainability.
Extracting methods involves identifying a block of code that performs a specific task and moving it into its own function. This not only makes the code more modular but also allows for reusability, as the extracted method can be called from multiple places within the codebase.
Eliminating nested conditionals is another effective way to reduce code complexity. Instead of having multiple levels of nested if statements, refactoring can simplify the logic by using guard clauses or early returns. This makes the code more readable and avoids the “arrow code” phenomenon, where the code stretches horizontally due to excessive indentation.
Improving Code Readability
Code readability is crucial for collaboration and future maintenance. Refactoring techniques such as meaningful variable naming, removing dead code, and adding comments can greatly enhance code readability and make it more accessible to other developers.
Clean and readable code not only improves team productivity but also reduces the time and effort required to debug and troubleshoot issues.
Meaningful variable naming is an essential aspect of code readability. By choosing descriptive and self-explanatory names for variables, developers can make the code more understandable at a glance. This helps in reducing cognitive load and makes it easier for other team members to comprehend the code.
Removing dead code, which refers to code that is no longer used or executed, is another important refactoring technique. Dead code can clutter the codebase and confuse developers, making it harder to understand the actual flow of the program. By removing unused code, the codebase becomes cleaner and more focused, leading to improved code readability.
Adding comments is a useful practice to provide additional context and explanations for complex or non-obvious parts of the code. Well-placed comments can help other developers understand the purpose and functionality of certain code sections, making it easier to collaborate and maintain the codebase.
Tools for Code Refactoring
Integrated Development Environments (IDEs)
Modern Integrated Development Environments (IDEs) are essential tools for developers, providing a comprehensive set of features to streamline the software development process. One of the key advantages of using an IDE is the built-in refactoring tools that automate common refactoring tasks.
These powerful tools assist developers in safely and efficiently restructuring their codebase without introducing errors. With just a few clicks, developers can refactor their code, improving its structure, readability, and maintainability.
IDEs often offer a wide range of refactoring features, including code navigation, extract method/variable, and rename refactorings. These features allow developers to easily navigate through their codebase, extract repetitive code into reusable methods or variables, and rename elements consistently throughout the project.
By leveraging these tools, developers can save valuable time and ensure that code refactoring is performed accurately. With automated refactoring capabilities, developers can focus on writing high-quality code and improving the overall design of their software.
Standalone Refactoring Tools
While IDEs provide excellent built-in refactoring tools, there are also standalone refactoring tools available that can be integrated into existing development workflows. These standalone tools offer advanced refactoring capabilities specific to different programming languages and frameworks.
Standalone refactoring tools provide additional flexibility and customization options, allowing developers to tailor their refactoring process to specific project requirements. These tools often come with a wide range of refactoring options, enabling developers to perform complex code transformations with ease.
Moreover, standalone refactoring tools often provide detailed analysis and suggestions for code improvements. They can identify potential code smells, performance bottlenecks, and other issues that can be addressed through refactoring. By utilizing these tools, developers can optimize their codebase, enhancing its performance and maintainability.
Integrating standalone refactoring tools into the development workflow can boost productivity and code quality. These tools empower developers to refactor their code efficiently, resulting in cleaner, more maintainable codebases.
Challenges and Solutions in Code Refactoring
Dealing with Large Code Bases
Refactoring large code bases can be challenging due to the complexity and interdependencies between different components. It requires a careful and systematic approach to ensure that the code remains maintainable and efficient.
One solution to this challenge is to break down the refactoring process into manageable units. By focusing on one module or feature at a time, developers can better understand the code and make targeted improvements. This approach not only reduces the complexity but also allows for easier testing and validation of the changes made.
Another important aspect of refactoring large code bases is the need for comprehensive automated tests. Writing these tests before starting the refactoring process can help ensure that the code remains functional throughout the changes. It provides a safety net that allows developers to catch any regressions or unintended side effects early on, minimizing the impact on the overall system.
Managing Refactoring Risks
Every refactoring effort carries the risk of introducing new bugs or breaking existing functionality. It is essential to have a well-defined strategy in place to mitigate these risks and ensure a successful refactoring process.
One effective way to manage refactoring risks is to maintain a comprehensive suite of automated tests that cover the codebase. These tests should be designed to detect any regressions or issues that may arise during the refactoring process. By running these tests regularly and addressing any failures promptly, developers can catch potential problems early on and prevent them from impacting the stability of the system.
In addition to automated tests, adhering to refactoring best practices is crucial. This includes following established patterns and guidelines, such as the SOLID principles, to ensure that the code remains clean, maintainable, and extensible. Regular code reviews and seeking feedback from team members can also help identify potential risks and provide valuable insights for improvement.
Overall, successfully managing the challenges of code refactoring requires a combination of careful planning, targeted improvements, and a strong focus on maintaining the functionality and stability of the codebase. By breaking down the process, writing comprehensive tests, and following best practices, developers can confidently tackle refactoring tasks and improve the overall quality of the code.
The Impact of Code Refactoring on Software Quality
Enhancing Software Maintainability
By improving code quality and reducing code complexity, refactoring enhances software maintainability. Clean and well-structured code is easier to understand, modify, and extend, leading to decreased maintenance costs and increased developer efficiency.
When code is refactored, it undergoes a transformation that not only improves its readability but also makes it more modular. This modular structure allows developers to isolate and fix bugs more easily, reducing the time and effort required for troubleshooting. Additionally, refactoring helps identify and eliminate redundant code, reducing the risk of introducing errors during future modifications.
Moreover, refactoring helps prevent the accumulation of technical debt, enabling development teams to deliver high-quality software consistently. Technical debt refers to the additional work that needs to be done in the future due to shortcuts or compromises made during the development process. By regularly refactoring code, developers can proactively address technical debt, ensuring that the software remains maintainable and adaptable in the long run.
Boosting Software Performance
Code refactoring can significantly impact software performance. By optimizing algorithms, improving data structures, and eliminating performance bottlenecks, developers can enhance the speed and efficiency of their applications.
During the refactoring process, developers can identify and replace inefficient algorithms with more efficient ones. This can lead to significant performance improvements, especially in resource-intensive tasks such as data processing or complex calculations. Additionally, refactoring allows developers to fine-tune data structures, ensuring that they are optimized for the specific requirements of the software.
Refactoring also allows developers to leverage new techniques or technologies that emerge over time, ensuring that the software remains up to date and performs optimally. As technology advances, new programming paradigms, libraries, or frameworks may become available, offering better performance or improved functionality. By periodically refactoring the codebase, developers can take advantage of these advancements, keeping the software competitive and responsive to user needs.
Conclusion: The Long-Term Benefits of Code Refactoring
In conclusion, code refactoring is not merely an optional step in the software development process but an essential practice for building high-quality, maintainable software. It allows developers to improve code readability, reduce complexity, and boost performance, resulting in a more efficient and sustainable codebase.
By adhering to the principles of code refactoring, utilizing the available tools, and overcoming challenges through structured approaches, development teams can reap the long-term benefits of improved software quality, enhanced maintainability, and increased productivity.
So, embrace code refactoring as an integral part of your software development journey and unlock its transformative power!
Transform Your Team’s Collaboration with Teamhub
Ready to take your team’s productivity to the next level? Teamhub is the collaboration platform you’ve been waiting for. With our intuitive, centralized hub, your small team can streamline Projects and Documentation, making code refactoring and other development processes more efficient than ever. Join the thousands of companies enhancing their workflow with Teamhub. Start your free trial today and experience the power of a single hub for your entire team.