LIMITED TIME OFFER
Replace all of these

with a single tool for just $49 per month for your entire team
UNLIMITED USERS
UNLIMITED PROJECTS
UNLIMITED CHATS
UNLIMITED DOCS
UNLIMITED STORAGE
AND MORE..
Understanding Design Patterns in Software Development
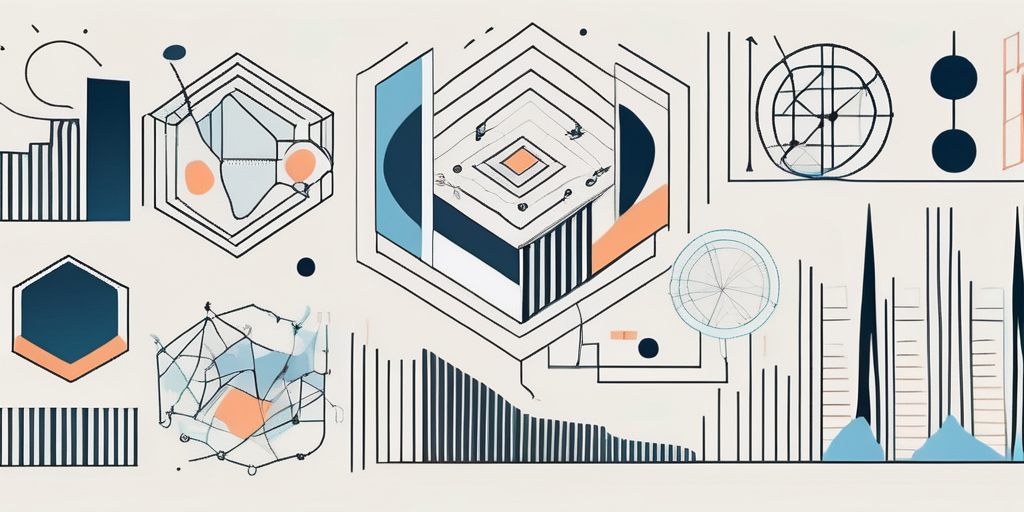
Design patterns play a crucial role in software development. They provide a proven solution to commonly occurring problems and help in creating flexible, reusable, and maintainable code. By understanding and utilizing design patterns effectively, developers can enhance the overall quality of their software.
The Importance of Design Patterns in Software Development
Design patterns offer several advantages in software development. One key benefit is the enhancement of flexibility and reusability in code. When design patterns are utilized, software components can be easily modified and adapted to meet changing requirements. This is particularly important in today’s fast-paced technological landscape, where software systems need to evolve and scale rapidly to keep up with the demands of users and market trends.
Moreover, design patterns promote code reuse, as developers can utilize existing patterns in new projects, saving time and effort. By leveraging proven solutions to common problems, developers can build upon the collective knowledge and experience of the software development community. This not only accelerates the development process but also reduces the likelihood of introducing errors or reinventing the wheel.
Another significant advantage of design patterns is the improvement in code readability and maintenance. By following established patterns, developers can create code that is clear and easy to understand. This makes it more straightforward for other developers to maintain and debug the code, reducing the risk of errors and increasing overall productivity.
Furthermore, design patterns provide a common language and framework for communication among developers. When a team of developers is familiar with design patterns, they can easily discuss and collaborate on software design decisions. This shared understanding facilitates effective teamwork, reduces misunderstandings, and promotes consistency in the codebase.
Additionally, design patterns can contribute to the scalability and performance of software systems. By employing patterns such as the Singleton or Observer pattern, developers can optimize resource usage and minimize bottlenecks. This can result in improved system performance, reduced response times, and enhanced user experience.
In conclusion, design patterns play a crucial role in software development by enhancing flexibility, promoting code reuse, improving code readability and maintenance, facilitating effective communication among developers, and contributing to the scalability and performance of software systems. By incorporating design patterns into their development practices, software engineers can build robust, maintainable, and efficient software solutions that meet the ever-changing needs of users and businesses.
The Basic Concepts of Design Patterns
Design patterns are a set of reusable solutions to common software problems. They provide a way to categorize and communicate software design best practices. Understanding the definition, purpose, and key principles of design patterns is crucial for developers to effectively utilize them in their projects.
Definition and Purpose of Design Patterns
Design patterns are general solutions to recurring problems in software design. They serve as guidelines for software development, offering proven solutions to issues commonly encountered during the development process. Design patterns are not specific to a particular programming language or technology but provide a higher-level approach to solving problems.
Let’s dive deeper into the purpose of design patterns. By utilizing design patterns, developers can achieve several benefits. Firstly, design patterns promote code reusability, allowing developers to save time and effort by leveraging existing solutions to common problems. This not only speeds up the development process but also enhances the overall quality of the software.
Furthermore, design patterns improve code maintainability and readability. By following established patterns, developers can create code that is easier to understand and modify. This is especially important when working on large-scale projects with multiple team members, as it ensures a consistent and cohesive codebase.
Key Principles of Design Patterns
Design patterns are founded on several key principles, such as abstraction, encapsulation, and separation of concerns. Abstraction allows developers to focus on essential features and hide unnecessary complexity. By abstracting away implementation details, design patterns enable developers to create flexible and scalable software.
Encapsulation is another fundamental principle of design patterns. It helps in achieving modularity and information hiding, ensuring that each component has a well-defined interface. This promotes code reusability and reduces the impact of changes in one component on the rest of the system.
Lastly, the principle of separation of concerns aims to create loosely coupled components that can be modified independently without impacting the overall system. By dividing the software into distinct modules, each responsible for a specific functionality, design patterns enable developers to manage complexity and enhance maintainability.
These key principles form the foundation of design patterns and guide developers in creating robust and well-structured software. By adhering to these principles, developers can ensure that their code is flexible, maintainable, and scalable.
Different Types of Design Patterns
Design patterns can be classified into three main categories: creational, structural, and behavioral patterns. These categories provide different strategies for creating objects, organizing classes, and defining communication between objects.
Creational design patterns focus on the creation of objects. They provide mechanisms for creating objects in a way that is decoupled from the specific classes used. This allows for flexibility and reusability in object creation. One example of a creational design pattern is the Singleton pattern. The Singleton pattern ensures the existence of only one instance of a class, making it useful in scenarios where only one instance of a class is needed throughout the entire application. Another example is the Factory pattern, which provides an interface for creating objects without specifying their concrete classes. This allows for the creation of objects based on certain conditions or parameters, making the code more flexible and maintainable.
Structural design patterns, on the other hand, deal with the composition of classes and objects to form larger structures. They help in organizing classes in a flexible and efficient manner. One commonly used structural design pattern is the Adapter pattern. The Adapter pattern allows incompatible interfaces to work together by acting as a bridge between them. It is particularly useful when integrating existing code with new code or when working with third-party libraries that have different interfaces. Another example is the Composite pattern, which treats individual objects and groups of objects uniformly. This pattern allows you to treat a single object or a group of objects in the same way, simplifying the code and making it more maintainable.
Behavioral design patterns focus on communication and interaction between objects. They provide solutions for controlling the behavior of objects and the flow of data between them. One example of a behavioral design pattern is the Observer pattern. The Observer pattern defines a one-to-many dependency relationship between objects, where changes in one object are automatically propagated to other dependent objects. This pattern is commonly used in event-driven systems or when implementing publish-subscribe mechanisms. Another example is the Strategy pattern, which allows interchangeable algorithms to be selected at runtime. This pattern is useful when you want to provide multiple algorithms for performing a specific task and allow the client to choose the most appropriate one at runtime.
Implementing Design Patterns in Software Development
Implementing design patterns requires a systematic approach. By following the steps outlined below, developers can successfully incorporate design patterns into their software projects:
Steps to Implementing a Design Pattern
- Identify the problem: Understand the specific problem you are trying to solve and determine if a design pattern is appropriate.
- Choose the appropriate pattern: Select the design pattern that best addresses the problem at hand.
- Study the pattern: Familiarize yourself with the pattern and understand its purpose, structure, and participants.
- Adapt the pattern: Tailor the pattern to fit your specific requirements, making any necessary modifications or additions.
- Implement the pattern: Apply the pattern in your code, ensuring that the design principles are followed.
- Test and refine: Validate the implementation of the pattern through rigorous testing and iterate on improvements if necessary.
Common Mistakes in Implementing Design Patterns
- Applying patterns unnecessarily: Design patterns should only be used when they provide a clear benefit. Avoid using patterns for the sake of using patterns.
- Overly complex solutions: Sometimes, developers may overcomplicate their code by trying to fit multiple patterns into a single solution. Keep the code as simple as possible.
- Ignoring context and trade-offs: Design patterns should be applied based on the specific context and trade-offs of the project. Consider factors such as performance, scalability, and maintainability.
- Lack of understanding: Implementing design patterns without a thorough understanding of their purpose and principles can lead to incorrect usage and potential issues.
While following the steps and avoiding common mistakes is crucial, it is also important to understand the benefits of implementing design patterns in software development. Design patterns provide reusable solutions to commonly occurring problems, allowing developers to save time and effort. By incorporating design patterns, developers can enhance the maintainability, flexibility, and scalability of their code.
Moreover, design patterns promote a structured and organized approach to software development. They provide a common language and framework for communication among developers, making it easier to understand and collaborate on complex projects. Design patterns also contribute to code readability and comprehensibility, as they encapsulate proven solutions and best practices.
The Role of Design Patterns in Agile Development
Agile development methodologies, such as Scrum and Kanban, can greatly benefit from the use of design patterns. These methodologies emphasize adaptability, collaboration, and iterative development, making design patterns a natural fit.
Design Patterns in Scrum
In Scrum, design patterns can help in creating flexible and maintainable code. By applying design patterns during the sprint planning phase, developers can anticipate and address potential design challenges early on, reducing the risk of delays or rework. Design patterns also support the agile principle of continuous integration by promoting code reuse and modularity.
Let’s take a closer look at one specific design pattern commonly used in Scrum: the Observer pattern. This pattern allows objects to establish a one-to-many relationship, where multiple observers are notified when a subject’s state changes. This can be particularly useful in Scrum, where cross-functional teams collaborate on a shared product backlog. By implementing the Observer pattern, teams can ensure that changes made by one team member are immediately communicated to others, promoting transparency and facilitating effective collaboration.
Design Patterns in Kanban
Kanban focuses on visualizing and optimizing the flow of work. Design patterns can aid in achieving efficiency in development processes by providing established solutions to common problems. By utilizing design patterns, development teams can improve collaboration and ensure a consistent approach to problem-solving.
One design pattern commonly used in Kanban is the Factory pattern. This pattern provides an interface for creating objects, allowing teams to encapsulate the object creation process and provide a consistent way of creating different types of objects. In Kanban, where teams often work on multiple projects simultaneously, the Factory pattern can help streamline the creation of different work items, ensuring that they adhere to predefined standards and reducing the risk of errors or inconsistencies.
Another design pattern that can be beneficial in Kanban is the Decorator pattern. This pattern allows for the dynamic addition of new behaviors to an object without altering its structure. In the context of Kanban, where work items often go through different stages and may require additional functionalities, the Decorator pattern can be used to add new features or modify existing ones without disrupting the overall flow of work. This promotes flexibility and adaptability, key principles of Kanban.
Future Trends in Design Patterns
As technology continues to evolve, the application of design patterns is expanding into new areas. Two emerging fields in which design patterns are gaining traction are cloud computing and machine learning/artificial intelligence.
The world of cloud computing is rapidly growing, with businesses and individuals alike relying on the flexibility and scalability it offers. Design patterns play a crucial role in addressing the unique challenges that come with cloud computing. For instance, the Circuit Breaker pattern is a valuable tool in ensuring the resilience and fault-tolerance of distributed systems in the cloud. By monitoring the health of services and preventing cascading failures, this pattern helps maintain the stability of cloud-based applications.
Another design pattern that proves invaluable in the realm of cloud computing is the Saga pattern. As cloud environments become increasingly complex, maintaining consistency across distributed transactions becomes a challenge. The Saga pattern provides a solution by breaking down long-running transactions into smaller, more manageable steps. This allows for better fault recovery and ensures that the system remains in a consistent state, even in the face of failures.
While cloud computing offers a wealth of opportunities, the rise of machine learning and artificial intelligence presents a whole new frontier for design patterns. These cutting-edge technologies require robust and adaptable systems to process vast amounts of data and make intelligent decisions. Design patterns can guide developers in building such systems.
The Observer pattern, for example, can be applied to create efficient and scalable machine learning pipelines. By decoupling the data processing and model training stages, this pattern allows for parallelization and improves overall system performance. Additionally, the Strategy pattern proves useful in the context of machine learning and AI, as it enables developers to easily switch between different algorithms or approaches, depending on the problem at hand.
As the field of machine learning and AI continues to evolve, design patterns will play a crucial role in shaping the development of intelligent systems. From data processing to model selection and system interaction, these patterns provide a solid foundation for building efficient and adaptable machine learning and AI applications.
Conclusion
Understanding design patterns is crucial for software developers aiming to create high-quality, flexible, and maintainable code. By implementing design patterns correctly, developers can enhance the overall efficiency of their software development process and adapt to evolving technological trends.
Remember, design patterns are not a one-size-fits-all solution but rather a set of guidelines. It is essential to analyze the specific requirements of each project and select the most appropriate design patterns accordingly. With a solid understanding of design patterns and their application, developers can truly unlock their potential in software development.
As you continue to explore the power of design patterns in software development, consider taking your team’s collaboration to the next level with Teamhub. Our platform is built to streamline your projects and documentation, ensuring that your development process is as efficient and integrated as possible. Embrace the future of teamwork and join the thousands of companies enhancing their productivity with Teamhub. Start your free trial today and experience a centralized hub that transforms the way your team operates.